How to consume API's with Axios in React.js Apps
- David Gonzales
- Nov 19, 2020
- 2 min read
Updated: Jan 3, 2023
Promise-based HTTP client for the browser and node.js ⬢
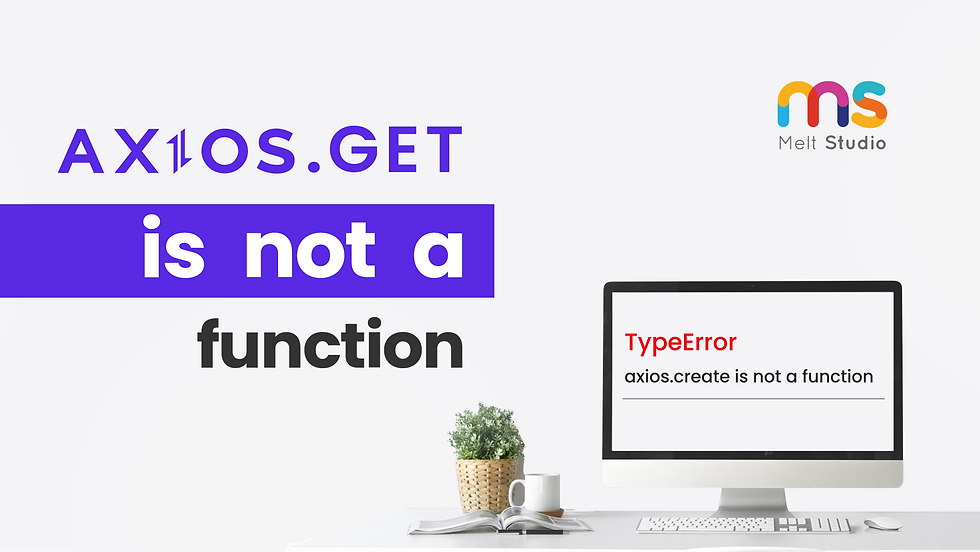
Axios is a lightweight HTTP Client based on promises.
No matter what framework is used, Axios is always compatible. Although it is outsourced and does not come integrated with javascript, like the Fetch API, the Axios library directly provides us with a response to HTTP requests. We only have to tell it that we want the response data, which makes it a strong competitor to fetch.

In short, Axios makes it easy to perform HTTP requests in apps. Other reasons to use Axios:
Automatically transforms data unlike fetch API
Less verbose (easy to write)
Easier to work with

How to use Axios?
We created a simple react app for this example. You need to install Node.js and follow the steps:
Install Axios inside the project folder app:
npm install axios
Import Axios into the component to use:
import axios from 'axios';
Define a const to store the HTTP response, this last one is precisely written: calling the Axios function and then, any of the methods of different HTTP verbs.
const getNameAxios = async () => {
try {
const resGet = await axios.get('https://jsonplaceh.../posts')
console.log(resGet)
} catch (error) {
console.log(error);
}
}
getNameAxios(6);
A GET to: https://jsonplaceholder.typicode.com/posts
ℹ️ Note: axios('https://...') is same thing as: axios.get('https://...')
Run the project:
npm start
Retrieving data from Axios is asynchronous because you basically cannot know how long it takes for it to retrieve the data over the network. And this kind of situation is what async/await is for.
As you can see, we are waiting for the request to finish, we are using the GET() method to make a request, and the return result doesn’t need to be converted to JSON:
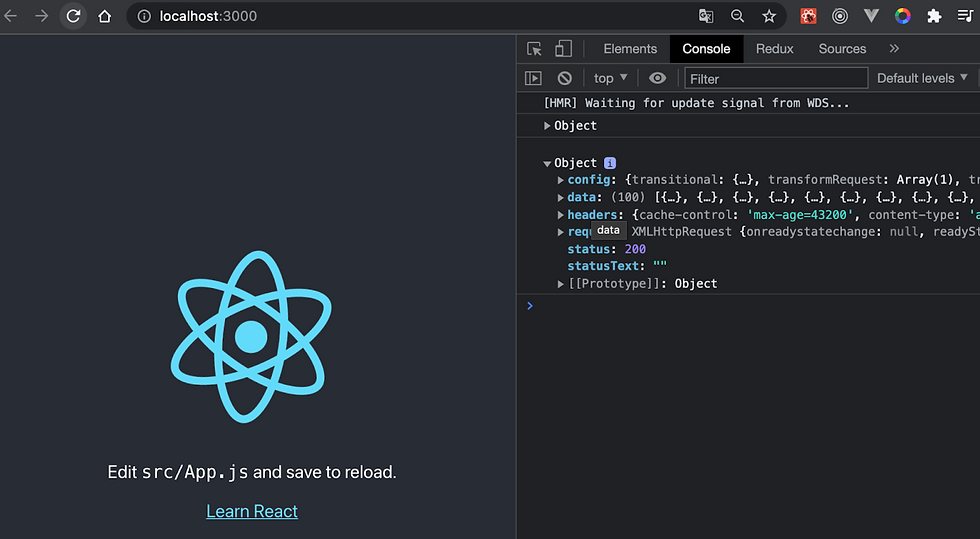
Axios HTTP Methods
For convenience, Axios also provides a set of shorthand methods or aliases that have been supplied for performing all supported HTTP request methods.
axios.request(config)
axios.get(url[, config])
axios.delete(url[, config])
axios.head(url[, config])
axios.options(url[, config])
axios.post(url[, data[, config]])
axios.put(url[, data[, config]])
axios.patch(url[, data[, config]])
Instance methods
The available instance methods and the specified config will be merged with the instance config.
axios#request(config)
axios#get(url[, config])
axios#delete(url[, config])
axios#head(url[, config])
axios#options(url[, config])
axios#post(url[, data[, config]])
axios#put(url[, data[, config]])
axios#patch(url[, data[, config]])
axios#getUri([config])
Review the next example:
If there is an error with the player, you can watch the video at the following LINK.
Advantage of Axios over Fetch API
Supports older browsers
Supports upload progress
Has built-in CSRF protection
Has a way to abort a request
Has a way to set a response timeout
Performs automatic JSON data transformation.
It has an interceptors API to edit requests and responses
The amount of code lines that are written using Axios is visibly less.
Axios has a fast and easy-to-use API for most HTTP uses. With this, you can reproduce the key features of the Axios library using the fetch() method of web browsers. A request example:
Using Axios:
axios.get('url')
.then((response) => {
console.log(response);
})
.catch((response) => {
console.log(error);
});
Without Axios:
let xhr = new XMLHttpRequest();xhr.open('GET', 'url', true);xhr.setRequestHeader('Content-type', 'application/json');
xhr.onreadystatechange = () => {
if (xhr.readyState === 4 && xhr.status === 200) {
(perform some code);
} else {
(handle error)
}
};
xhr.send();
Ultimately, this API allows client-side protection against XSRF. It also has the ability to cancel requests.
Browser support
When it comes to browser support, Axios is very reliable. Even older browsers such as IE 11 work well with Axios.

To know more about Axios, review the documentation.
_________________________________________________________________________________________
¡Thanks for reading!
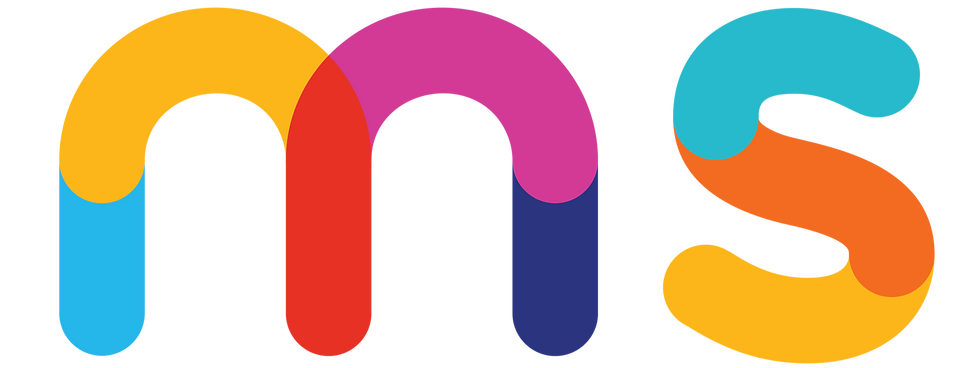
📍 Connect with us on instagram👇
Comments